Hi,
Animated gifs:
They are not supported, if you want to use animated textures, you'll have to make a single texture strip including multiple images, then animate texture translate to decal your texture in time.
Bullets:
There is a way to do it by script, but I should say that it will be more easy and optimized to use a c++ game plugin.
Look at Maratis/Examples/Demos/scripts/follow.lua to have a look on a more complex lua script to move objects.
Later, I'll try to give you a better example.
About shaders :
Create a custom shader will be the best to have your reflexion effect, in this case, as Kralle suggested, an envmap will be good enough.
A spherical envmap like this one for example :

You can link shader using Maratis Blender export :
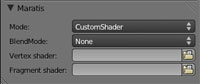
You can access each texture slot with your custom shader
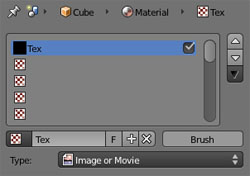
To create shaders, you can just use a text editor, as Maratis is updating assets in realtime, each time you will modify and save your shader, the result will automatically appear on Maratis.
To create a reflexion using a shader, you can use the normals as uv coord, something like :
VERTEX SHADER:
attribute vec3 Vertex;
attribute vec3 Normal;
uniform mat4 ModelViewMatrix;
uniform mat4 ProjModelViewMatrix;
uniform mat4 NormalMatrix;
varying vec3 position, normal;
void main(void)
{
normal = (NormalMatrix * vec4(Normal, 1.0)).xyz;
position = (ModelViewMatrix * vec4(Vertex, 1.0)).xyz;
gl_Position = ProjModelViewMatrix * vec4(Vertex, 1.0);
}
FRAGMENT SHADER:
uniform sampler2D Texture[8];
varying vec3 position, normal;
void main(void)
{
vec3 N = normalize(normal);
vec4 reflection = texture2D(Texture[0], N.xy);
gl_FragColor = reflection;
}
This example is only showing how to make an envmap, for a full shader including texture, diffuse, normal map, you can have a look to the standard Maratis shaders, located in the source distribution : Maratis/Common/MRenderers/MStandardShaders.h
When I'll have some time, I'll try to give a standard shader that can be used as a start.
Anaël.